Lisp »Tips 'n Tricks
»Frattali »1 »2 »3 »4 »5 »6 »7
LRNZ3
Due lisp per disegnare l'attrattore strano di Lorenz (e l'effetto farfalla) usando al posto del comando "punto" l'entità linea.
;|
LRNZ3.LSP (C) 2005 by Claudio Piccini.
www.cg-cad.com
Attrattore strano di Lorenz
dx/dt=-ax+ay
dy/dt=b*x-y-z*x
dz/dt=-cz+xy
|;
(defun lorenz3 ( / steps ; iterazioni
a b c ; costanti
dt ; step temporale
x y z
xx yy zz
i p0
)
(setq x 1
y 1
z 1
dt 0.02
)
(setq a (getreal "\n a? [5] "))
(if (= a nil)(setq a 5))
(setq b (getreal "\n b? [15] "))
(if (= b nil)(setq b 15))
(setq c (getreal "\n c? [1] "))
(if (= c nil)(setq c 1))
(setq steps (getint "\n steps? [10000] "))
(if (= steps nil)(setq steps 10000))
(setq p0 (getpoint "\ clicca un punto nel disegno..."))
(setq i 0)
(while (<= i steps)
(setq xx (+ (- x (* a x dt))(* a y dt)))
(setq yy (- (+ y (* b x dt))(* y dt)(* z x dt)))
(setq zz (+ (- z (* c z dt))(* x y dt)))
(command "_line"
(list (+ x (car p0))(+ y (cadr p0)) z)
(list (+ xx (car p0))(+ yy (cadr p0)) zz)
""
)
(setq x xx)
(setq y yy)
(setq z zz)
(setq i (1+ i))
)
)
(defun c:lrnz3 ( / snapp )
(setvar "cmdecho" 0)
(setq snapp (getvar "osmode"))
(command "_osnap" "_non")
(lorenz3)
(setvar "osmode" snapp)
(command "_redraw")
(setvar "cmdecho" 1)
(princ)
)
;;;eof
|
Test del lisp
Command: lrnz3
a? [5] Invio
b? [15] Invio
c? [1] Invio
steps? [10000] Invio
clicca un punto nel disegno...
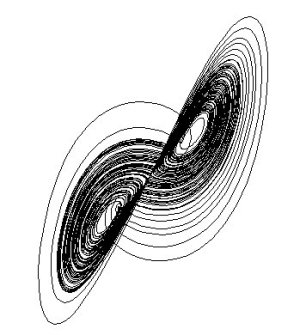
LRNZ4
;|
LRNZ4.LSP (C) 2005 by Claudio Piccini.
www.cg-cad.com
Disegna 2 attrattori strani di Lorenz
per simulare l'effetto farfalla
|;
(defun lorenz4 ( / steps ; iterazioni
a b c ; costanti
dt ; step temporale
x y z xx yy zz ; 1 attrattore
x1 y1 z1 xx1 yy1 zz1 ; 2 attrattore
i p0
)
(setq x 1.0000000
y 1.0000000
z 1.0000000
x1 1.0000001
y1 1.0000001
z1 1.0000001
dt 0.02
)
(setq a (getreal "\n a? [5] "))
(if (= a nil)(setq a 5))
(setq b (getreal "\n b? [15] "))
(if (= b nil)(setq b 15))
(setq c (getreal "\n c? [1] "))
(if (= c nil)(setq c 1))
(setq steps (getint "\n steps? [10000] "))
(if (= steps nil)(setq steps 10000))
(setq p0 (getpoint "\ clicca un punto nel disegno..."))
(setq i 0)
(while (<= i steps)
;|
disegna il primo attrattore
in rosso
|;
(command "_color" 1)
(setq xx (+ (- x (* a x dt))(* a y dt)))
(setq yy (- (+ y (* b x dt))(* y dt)(* z x dt)))
(setq zz (+ (- z (* c z dt))(* x y dt)))
(command "_line"
(list (+ x (car p0))(+ y (cadr p0)) z)
(list (+ xx (car p0))(+ yy (cadr p0)) zz)
""
)
(setq x xx)
(setq y yy)
(setq z zz)
;|
disegna il secondo attrattore
in blu
|;
(command "_color" 5)
(setq xx1 (+ (- x1 (* a x1 dt))(* a y1 dt)))
(setq yy1 (- (+ y1 (* b x1 dt))(* y1 dt)(* z1 x1 dt)))
(setq zz1 (+ (- z1 (* c z1 dt))(* x1 y1 dt)))
(command "_line"
(list (+ x1 (car p0))(+ y1 (cadr p0)) z1)
(list (+ xx1 (car p0))(+ yy1 (cadr p0)) zz1)
""
)
(setq x1 xx1)
(setq y1 yy1)
(setq z1 zz1)
(setq i (1+ i))
)
)
(defun c:lrnz4 ( / snapp )
(setvar "cmdecho" 0)
(setq snapp (getvar "osmode"))
(command "_osnap" "_non")
(lorenz4)
(setvar "osmode" snapp)
(command "_redraw")
(setvar "cecolor" "BYLAYER")
(setvar "cmdecho" 1)
(princ)
)
;;;eof
|
Test del lisp
Command: lrnz4
a? [5] Invio
b? [15] Invio
c? [1] Invio
steps? [10000] Invio
clicca un punto nel disegno...
Particolare
Command: lrnz4
a? [5] Invio
b? [15] Invio
c? [1] 3.5
steps? [10000] Invio
clicca un punto nel disegno...
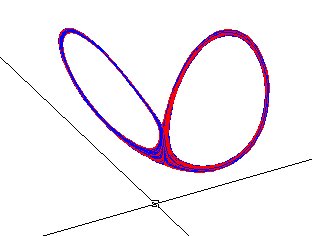
Command: lrnz4
a? [5] 3.5
b? [15] 10
c? [1] 3
steps? [10000] Invio
clicca un punto nel disegno...
...
Zero length line created at (237.0886, 98.7996, 9.0000)
; error: Function cancelled
Specify next point or [Undo]: *Cancel*
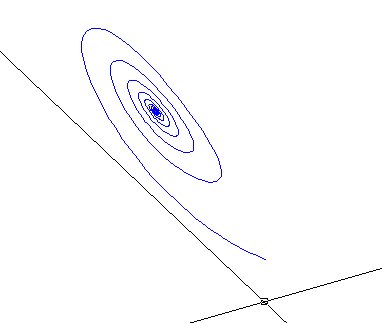
Lisp »Tips 'n Tricks
Ultimo Aggiornamento_Last Update: 25 Aprile 2005
|