Lisp »Tips 'n Tricks
»Funzioni ricorsive in AutoLISP
»1
| 2
| 3
| 4
| 5
| 6
| 7 a
, b
, c
, d
, e
>II
>III
>IV
>V
>VI
>VII
CESP.LSP
Con le funzioni ricorsive è possibile disegnare motivi decorativi, ad esempio questo lisp disegna un cespuglio.
;;;
;;; cesp.lsp - 9 Marzo 2004
;;; (C) 2004 by Claudio Piccini.
;;; www.cg-cad.com
;;;
;;; disegna un cespuglio
;;;
(defun myerror (s)
(if (/= s "Function cancelled")
(princ (strcat "\nError: " s))
)
(ripVar)
(princ)
)
(defun salVar ()
(setq orto (getvar "orthomode"))
(setq snapp (getvar "osmode"))
(setq snm (getvar "snapmode"))
(setq piano (getvar "clayer"))
)
(defun ripVar ()
(command "_redraw")
(setvar "cmdecho" 1)
(setvar "osmode" snapp)
(setvar "snapmode" snm)
(setvar "orthomode" orto)
(setvar "clayer" piano)
(setvar "cecolor" "BYLAYER")
(setq *error* olderr)
(princ)
)
; converte angolo da gradi in radianti
(defun g2r (a)
(* pi (/ a 180.0))
)
(defun disegna (lung ang)
(if (< lung n)
(progn
(setq p3 (polar p1 ang lung))
(command "_line" p1 p3 "")
(setq p1 (polar p1 ang lung))
)
(progn
(disegna (/ lung (sqrt 2.0))(+ (* ang -1)(g2r 15)))
(command "_color" "_green")
(disegna (/ lung (sqrt 2.0))(+ (* ang -1)(g2r 90)))
(command "_color" "_yellow")
(disegna (/ lung (sqrt 2.0))(- (* ang -1)(g2r 45)))
)
)
)
(defun c:cesp (/ p1 p2 ang lung n
olderr orto snapp snm piano
)
(setq olderr *error* *error* myerror)
(setvar "cmdecho" 0)
(salVar)
(command "_osnap" "_non")
(setq p1 (getpoint "\nprimo punto:"))
(setq p2 (getpoint p1 "\nsecondo punto: "))
;tenersi bassi con il numero di iterazioni
(setq n (getint "\nnumero iterazioni: "))
(setq ang (angle p1 p2))
(setq lung (distance p1 p2))
(setq n (/ lung n))
(disegna lung ang)
(ripVar)
)
;;;eof
|
Test del LISP
Command: cesp
primo punto:
secondo punto:
numero iterazioni: 8
Command: cesp
primo punto:
secondo punto:
numero iterazioni: 12
STAR.LSP
; Star -- Draw a Star
; Original by Carl Guerin !!/11/92
;
; Modified by Claudio Piccini, www.cg-cad.com
; 9 Marzo 2004
(defun sstar (outrad n / count)
(if (and (> n 0)(> outrad inrad))
(progn
(command "_PLINE")
(setq Count 0)
(while (< Count Points)
(command (polar Center (* Count Incr) OutRad))
(if (= Count 0)
(command "_width" "0" "0")
)
(command (polar center (+ (* Count Incr) Incr2) InRad))
(setq Count (1+ Count))
)
(command "_close")
(sstar (- outrad dim) (- n 1))
)
)
)
; Star -- Draw a Star
(defun C:STAR ( / outrad inrad points incr incr2)
(setvar "cmdecho" 0)
(setq snapp (getvar "osmode"))
(command "_osnap" "_non")
(initget 1)
(setq Center (getpoint "\nCenter of Star: "))
(initget (+ 1 2 4))
(setq OutRad (getdist "\nOutside Radius: " Center))
(initget (+ 1 2 4))
(setq InRad (getdist "\nInside Radius: " Center))
(initget (+ 1 2 4))
(setq Points (getint "\nNumber of Points: "))
(while (< Points 2)
(prompt "\nMust have at least 2 points!\n")
(initget (+ 1 2 4))
(setq Points (getint "\nNumber of Points: "))
)
(setq Incr (/ (* 2 pi) Points))
(setq Incr2 (/ Incr 2))
(setq n (getint "\nNumero iterazioni: "))
(setq dim (/ outrad n))
(sstar outrad n)
(setvar "osmode" snapp)
(command "_redraw")
(setvar "cmdecho" 1)
(princ)
)
|
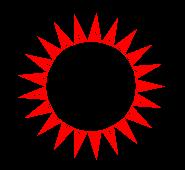
STAR2.LSP
; Star -- Draw a Star
; Original by Carl Guerin !!/11/92
;
; Modified by Claudio Piccini, www.cg-cad.com
; 9 Marzo 2004
(defun sstar (outrad n / count)
(if (> n 0)
(progn
(command "_PLINE")
(setq Count 0)
(while (< Count Points)
(command (polar Center (* Count Incr) OutRad))
(if (= Count 0)
(command "_width" "0" spes)
)
(command (polar center (+ (* Count Incr) Incr2) InRad))
(setq Count (1+ Count))
(if (= Count Points)
(command "_width" spes "0")
)
)
(command "_close")
(setq spes 0)
(command "_color" "_yellow")
(sstar (- outrad dim) (- n 1))
)
)
)
(defun C:STAR ( / outrad inrad points incr incr2)
(setvar "cmdecho" 0)
(setq snapp (getvar "osmode"))
(command "_osnap" "_non")
(initget 1)
(setq Center (getpoint "\nCenter of Star: "))
(initget (+ 1 2 4))
(setq OutRad (getdist "\nOutside Radius: " Center))
(initget (+ 1 2 4))
(setq InRad (getdist "\nInside Radius: " Center))
(initget (+ 1 2 4))
(setq Points (getint "\nNumber of Points: "))
(while (< Points 2)
(prompt "\nMust have at least 2 points!\n")
(initget (+ 1 2 4))
(setq Points (getint "\nNumber of Points: "))
)
(setq Incr (/ (* 2 pi) Points))
(setq Incr2 (/ Incr 2))
(setq n (getint "\nNumero iterazioni: "))
(setq spes (/ inrad 3))
(setq dim (/ outrad n))
(command "_color" "_red")
(sstar outrad n)
(command "_color" "_BYLAYER")
(setvar "osmode" snapp)
(command "_redraw")
(setvar "cmdecho" 1)
(princ)
)
|
Test del LISP
Command: star
Center of Star:
Outside Radius:
Inside Radius:
Number of Points: 100
Numero iterazioni: 100
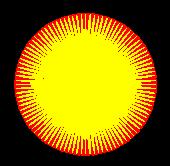
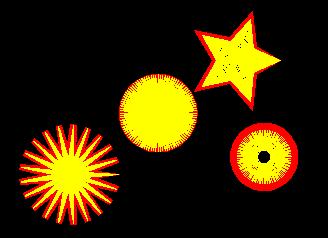
Lisp »Tips 'n Tricks
Ultimo Aggiornamento_Last Update: 9 Marzo 2004
|