Lisp »Tips 'n Tricks
»Funzioni ricorsive in AutoLISP »1 | 2 | 3 | 4 | 5 | 6 | 7
NP
Np è la traduzione in Autolisp di un programma in Pascal che dichiara se un numero è primo (divisibile solo per se stesso e per l'unità).
;;;
;;; np.lsp - 9 Febbraio 2004
;;; (C) by Claudio Piccini
;;; http://www.cg-cad.com/
;;;
;;; Traduzione in Autolisp di
;;; numpr.pas by Ali Qamar
;;; Description: Finds a prime number.
;;; The program is made recursively
;;;
(defun primo (a)
(cond
((= a 1)
(princ "\nIl numero e' primo")
(princ)
)
((= (rem num a) 0) ; num mod a=0
(princ "\nIl numero non e' primo")
(princ)
)
((/= a 1)
(primo (- a 1))
)
)
)
(defun c:np (/ num y)
(initget (+ 1 2 4))
(setq num (getint "\nInserisci un numero: "))
(setq y (/ num 2))
(if (= num 1)
(progn
(princ "\nIl numero e' primo")
(princ)
)
(princ (primo y))
)
)
;;;eof
|
{
Name: Ali Qamar
Email: yaarali@hotmail.com
Language: Pascal
Category: Miscellaneous
Description: Finds a prime number.
The program is made recursively
}
program Numpr;
var
num, y : integer;
procedure prime (a : integer);
begin
if a=1 then
writeln('The number is prime ')
else if
num mod a=0 then
writeln('The number is not prime')
else if a<>1 then
prime(a-1);
end;
begin
write('Enter a number : ');
readln(num);
y := num div 2;
if num=1 then
writeln('The number is prime')
else
prime(y);
readln;
end.
numpr.zip
|
Test del LISP
Provo np.lsp con i numeri: 1, 2, 3, 4, 5, 6, 7
Command: np
Inserisci un numero: 1
Il numero e' primo
Command: np
Inserisci un numero: 2
Il numero e' primo
Command: np
Inserisci un numero: 3
Il numero e' primo
Command: np
Inserisci un numero: 4
Il numero non e' primo
Command: np
Inserisci un numero: 5
Il numero e' primo
Command: np
Inserisci un numero: 6
Il numero non e' primo
Command: np
Inserisci un numero: 7
Il numero e' primo
FRT1
Frt1 è un Lisp che disegna un quadrato di dimensione 2r centrato nel punto p1(x,y). La funzione ricorsiva permette di generare un frattale.
L'algoritmo di base è il seguente:
star(int x, int y, int r)
{
if (r > 0)
{
star(x-r,y+r,r/2);
star(x+r,y+r,r/2);
star(x-r,y-r,r/2);
star(x+r,y-r,r/2);
box(x,y,r);
}
}
Da: "Algoritmi in C++", R. Sedgewick Ed. Addison-Wesley
;;;
;;; frt1.lsp - 9 Febbraio 2004
;;; (C) by Claudio Piccini
;;; http://www.cg-cad.com/
;;;
;;; Disegna un frattale
;;;
(defun myerror (s)
(if (/= s "Function cancelled")
(princ (strcat "\nError: " s))
)
(ripVar)
(princ)
)
(defun salVar ()
(setq orto (getvar "orthomode"))
(setq snapp (getvar "osmode"))
(setq snm (getvar "snapmode"))
(setq piano (getvar "clayer"))
)
(defun ripVar ()
(command "_redraw")
(setvar "cmdecho" 1)
(setvar "osmode" snapp)
(setvar "snapmode" snm)
(setvar "orthomode" orto)
(setvar "clayer" piano)
(setq *error* olderr)
(princ)
)
(defun star (x y r)
(if (> r 0)
(progn
(star (- x r)(+ y r)(/ r 2))
(star (+ x r)(+ y r)(/ r 2))
(star (- x r)(- y r)(/ r 2))
(star (+ x r)(- y r)(/ r 2))
(setq p1 (list x y 0))
(command "_polygon" 4 p1 "" r)
)
)
)
(defun c:frt1 (/ p1 x y r)
(setq olderr *error* *error* myerror)
(setvar "cmdecho" 0)
(salVar)
(command "osnap" "_non")
(setq p1 (getpoint "\nClicca un punto:"))
(initget (+ 2 4))
(setq r (getint "\nRaggio del quadrato (10): "))
(if (= r nil)
(setq r 10)
)
(setq x (car p1))
(setq y (cadr p1))
(star x y r)
(ripVar)
)
;;;eof
|
Test del LISP
Raggio = 10.
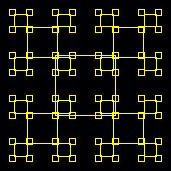
Lisp »Tips 'n Tricks
Ultimo Aggiornamento_Last Update: 9 Febbraio 2004
|