Lisp »Tips 'n Tricks
»Simmetrie »1 »2 »3 »4 »5 »6 »7
TRAP2 variante n.2
Questa variante del lisp trap2 genera una tassellatura di punti colorati in modo casuale da una generica formula f(x)[+-/*]f(y).
;|
TRAP2.LSP variante n.2
(C) 2005 by Claudio Piccini.
www.cg-cad.com
Lisp per disegnare tassellature
Traduzione in autolisp dell'algoritmo 'Egg Tile Generator'
in 'Computers, Pattern, Chaos and Beauty' di C.A. Pickover
2001, Dover Publications, Inc.
|;
(defun tass_f (funz / dim beta1 beta2 gamma alpha
i j x y z
sd sx
)
(setq sd 0.0)
(initget (+ 2 4)) ; non 0, non negativo
(setq dim (getreal "\nDim? [100] "))
(if (= dim nil)(setq dim 100))
; beta1
(setq beta1 (getint "\nBeta1? [-11] "))
(if (= beta1 nil)(setq beta1 -11))
; beta2
(setq beta2 (getint "\nBeta2? [-12] "))
(if (= beta2 nil)(setq beta2 -12))
; gamma
(setq gamma (getint "\nGamma? [60] "))
(if (= gamma nil)(setq gamma 60))
; Alpha
(setq alpha (getint "\nAlpha? [10] "))
(if (= alpha nil)(setq alpha 10))
(setq i 1)
(while (<= i dim)
(setq j 1)
(while (<= j dim)
(setq x (+ beta1 (* gamma i)))
(setq y (+ beta2 (* gamma j)))
(setq z (* alpha (eval (read funz))))
(if (= (rem (fix z) 3) 0)
(progn
(setq sx (rn sd))
(setq sd sx)
(command "_color" (1+ (fix sx)))
(command "_point" (list i j))
)
)
(setq j (1+ j))
)
(setq i (1+ i))
)
)
;|
Genera un numero casuale compreso tra 0 e 6
Vedi il tutorial n.37 "Numeri casuali"
in AutoLISP Tips & Tricks Volume I
|;
(defun rn (sd)
(setq m 65521 b 15937 c 33503 sd
(rem (+ (* b sd) c) m)
)
(setq sd (* (/ sd m) 7))
)
(defun c:trap2 ( / snapp funz )
(setvar "cmdecho" 0)
(setq snapp (getvar "osmode"))
(command "_osnap" "_non")
(setq funz (getstring "\nFunzione (ad es. \"(+ (sin x)(sin y))\"): "))
(tass_f funz)
(setvar "osmode" snapp)
(command "_redraw")
(setvar "cecolor" "BYLAYER")
(setvar "cmdecho" 1)
(princ)
)
;;;eof
|
Test del lisp
Command: trap2
Funzione (ad es. "(+ (sin x)(sin y))"): "(* (sin x)(sin y))"
Dim? [100] Invio
Beta1? [-11] 10
Beta2? [-12] 3
Gamma? [60] 25
Alpha? [10] Invio
Funzione (ad es. "(+ (sin x)(sin y))"): "(* (sin x)(sin (log y)))"
Dim? [100] Invio
Beta1? [-11] 10
Beta2? [-12] 3
Gamma? [60] 25
Alpha? [10] Invio
Funzione (ad es. "(+ (sin x)(sin y))"): "(+ (log x)(log y))"
Dim? [100] Invio
Beta1? [-11] 10
Beta2? [-12] 3
Gamma? [60] 25
Alpha? [10] Invio
Analisi del lisp
(eval (read funz))
La funzione (eval espr) restituisce il risultato dell'espressione elaborata.
Esempi:
(eval 4.0)
4.0
(eval (abs -10))
10
(eval "(+ (sin x)(sin y))")
"(+ (sin x)(sin y))"
La funzione (read stringa) restituisce l'elemento di una stringa.
Esempi:
(read "hello")
HELLO
(read "hello there")
HELLO
(read "\"Hi Y'all\"")
"Hi Y'all"
(read "(a b c)")
(A B C)
(read "(a b c) (d)")
(A B C)
Command: (eval (read "(- (cos (* 2 pi))(cos pi))"))
2.0
TRAP2 variante n.3
Questa variante del lisp trap2 genera una tassellatura di punti colorati in modo casuale (con trama diversa ad ogni sessione del lisp) da una generica formula
f(x)[+-/*]f(y).
;|
TRAP2.LSP variante n.3
(C) 2005 by Claudio Piccini.
www.cg-cad.com
Lisp per disegnare tassellature
Traduzione in autolisp dell'algoritmo 'Egg Tile Generator'
in 'Computers, Pattern, Chaos and Beauty' di C.A. Pickover
2001, Dover Publications, Inc.
|;
(defun tass_f (funz / dim beta1 beta2 gamma alpha
i j x y z
)
(initget (+ 2 4)) ; non 0, non negativo
(setq dim (getreal "\nDim? [100] "))
(if (= dim nil)(setq dim 100))
; beta1
(setq beta1 (getint "\nBeta1? [-11] "))
(if (= beta1 nil)(setq beta1 -11))
; beta2
(setq beta2 (getint "\nBeta2? [-12] "))
(if (= beta2 nil)(setq beta2 -12))
; gamma
(setq gamma (getint "\nGamma? [60] "))
(if (= gamma nil)(setq gamma 60))
; Alpha
(setq alpha (getint "\nAlpha? [10] "))
(if (= alpha nil)(setq alpha 10))
(setq i 1)
(while (<= i dim)
(setq j 1)
(while (<= j dim)
(setq x (+ beta1 (* gamma i)))
(setq y (+ beta2 (* gamma j)))
(setq z (* alpha (eval (read funz))))
(if (= (rem (fix z) 3) 0)
(progn
(command "_color" (1+ (fix (rn))))
(command "_point" (list i j))
)
)
(setq j (1+ j))
)
(setq i (1+ i))
)
)
;|
Estrae un numero casuale da 0 a 6
Il seme (sd) e' inizializzato con la variabile DATE
in questo modo la serie di numeri casuali
e' diversa in ogni sessione del lisp.
Vedi il tutorial n.37 "Numeri casuali"
in AutoLISP Tips & Tricks Volume I
|;
(defun rn ( / m b c )
(if (not sd)
(setq sd (getvar "DATE"))
)
(setq m 65521
b 15937
c 33503
)
(setq sd (rem (+ (* b sd) c) m))
(setq sd (* (/ sd m) 7))
)
(defun c:trap2 ( / snapp sd funz )
(setvar "cmdecho" 0)
(setq snapp (getvar "osmode"))
(command "_osnap" "_non")
(setq funz (getstring "\nFunzione (ad es. \"(+ (sin x)(sin y))\"): "))
(tass_f funz)
(setvar "osmode" snapp)
(command "_redraw")
(setvar "cecolor" "BYLAYER")
(setvar "cmdecho" 1)
(princ)
)
;;;eof
|
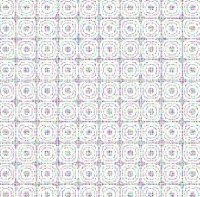
Lisp »Tips 'n Tricks
Ultimo Aggiornamento_Last Update: 10 Aprile 2005
|